Below, I have a code (which can be interpreted as pseudocode in this context) and output where I indicate where the “skip” appear.
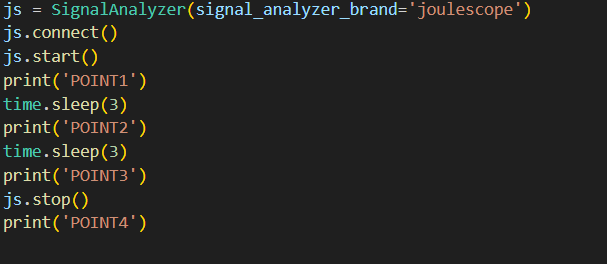
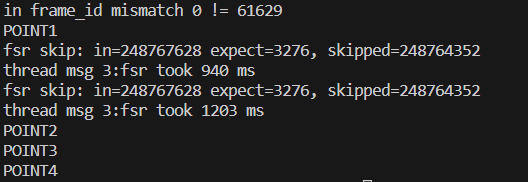
To me, it seems to be happening early in the process. I also detect this in the converted .csv file (from .jls) (Sampling frequency = 1000Hz)
time,current,voltage
0.0,-5.401671e-08,0.0024840143
0.001,-4.749745e-08,0.0025642468
0.002,-4.284084e-08,0.0025480436
0.003,-6.891787e-08,0.0025303029
0.004,-7.264316e-08,0.002540309
0.005,-6.053597e-08,0.0025325455
0.006,-7.264316e-08,0.0025331695
0.007,-7.636845e-08,0.0025186744
0.008,-7.264316e-08,0.0025588945
0.009000000000000001,-7.916242e-08,0.0025674133
0.01,-8.288771e-08,0.00256095
0.011,-8.195639e-08,0.002569857
0.012,-8.381903e-08,0.002550017
0.013000000000000001,-6.798655e-08,0.002543306
0.014,-6.053597e-08,0.0025718044
0.015,-6.61239e-08,0.0025843717
0.016,-6.239861e-08,0.002568148
0.017,-5.401671e-08,0.0025464306
0.018000000000000002,-4.9360096e-08,0.0025457004
0.019,-5.2154064e-08,0.0025195945
0.02,-5.2154064e-08,0.0025027487
0.021,-4.4703484e-08,0.0025146948
0.022,-3.8184226e-08,0.0025454294
0.023,-6.891787e-08,0.0025356747
0.024,-7.8231096e-08,0.002521269
0.025,-6.426126e-08,0.0025719907
0.026000000000000002,-7.357448e-08,0.0025738822
0.027,-7.7299774e-08,0.0025654603
0.028,-7.543713e-08,0.0026190989
0.029,nan,nan
0.03,nan,nan
0.031,nan,nan
0.032,nan,nan
0.033,nan,nan
0.034,nan,nan
0.035,nan,nan
0.036000000000000004,nan,nan
…continued…
431.681,nan,nan
431.682,nan,nan
431.683,nan,nan
431.684,nan,nan
431.685,nan,nan
431.68600000000004,nan,nan
431.687,nan,nan
431.688,nan,nan
431.689,nan,nan
431.69,nan,nan
431.69100000000003,nan,nan
431.692,nan,nan
431.693,nan,nan
431.694,nan,nan
431.695,-8.1025064e-08,0.0025817119
431.696,-7.8231096e-08,0.002595636
431.697,-7.7299774e-08,0.0026291562
431.69800000000004,-8.8475645e-08,0.0025607012
431.699,-6.61239e-08,0.0025456138
431.7,-6.053597e-08,0.002601483
431.701,-6.519258e-08,0.0025360603